equal
TypeScript-first deep equivalence comparison between two values
Equivalent comparison of Object data structures. It supports many built-in objects and can be compared with Date
, Array
or Object
.
The supported built-in objects are here
🔖 Table of Contents
✨ Features
- ⚡ Multi runtime support (
Deno
,Node.js
and Browsers) - 📚 Pure TypeScript and provides type definition
- ✅ Rambda's all test case is passed
- 🌎 Universal module, providing
ES modules
andCommonjs
- 📦 Optimized, super slim size
- 📄 TSDoc-style comments
Package name
Deno: equal
(deno.land, nest.land)
Node.js: lauqe
(npm)
⚡ Example
Primitive
equal('', '') // true
equal(NaN, NaN) // true
equal(0, 0) // true
equal(+0, 0) // true
equal(-0, 0) // true
equal(+0, -0) // true
equal(0n, 0n) // true
equal(undefined, undefined) // true
equal(null, null) // true
equal(undefined, null) // false
equal(true, false) // false
const symbol = Symbol("hello");
equal(symbol, symbol) // true
equal(Symbol('hello'), Symbol('hello')) // false
Object
equal({}, {}) // true
equal({ "": undefined }, { "": undefined }) // true
equal({ "": undefined }, { "": undefined, a: 1 }) // false
equal({ a: 1, b: undefined}, { b: undefined, a: 1}) // true
equal([], []) // true
equal([[[]]], [[[]]]) // true
equal([[{ a: [] }]], [{ a: [] }]) // true
equal(new Date("2000/1/1"), new Date("2000/1/1")) // true
equal(new Date("2000/1/1"), new Date("2000/1/1 00:00:01")) // false
equal(() => true, () => true) // true
equal(() => true, () => false) // false
equal(Error('hoge'), Error('hoge')) // true
equal(Error('hoge'), Error('huga')) // false
equal(TypeError('hoge'), TypeError('hoge')) // true
equal(Error('hoge'), TypeError('hoge')) // false
equal(RangeError('error'), ReferenceError('error')) // false
equal(SyntaxError('error'), URIError('error')) // false
equal(AggregateError([ Error("error"), TypeError("type error") ]), AggregateError([ Error("error"), TypeError("type error") ])) // true
equal(/s/, /s/) // true
equal(/s/, /t/) // false
equal(/s/gi, /s/gi) // true
equal(/s/gi, /s/gim) // false
equal(new String('hello'), new String('hello')) // true
equal(new Number(0), new Number(0)) // true
equal(new Boolean(true), new Boolean(true)) // true
equal(new Map([[1, 2], [3, 4]]), new Map([[3, 4], [1, 2]]) // true
equal(new Map([[new Map(), { a: 1 } ]), new Map([[new Map(), { a: 1 } ]) // true
equal(new Set(), new Set()) // true
equal(new Set([[], {}, new Map(), new Set()]), new Set([[], {}, new Map(), new Set()])) // true
📝 API
Type definition
declare const equal: <T, U extends T>(a: T, b: U) => boolean
Parameter | Description |
---|---|
a |
Any value |
b |
Any value |
=>
Return true
if the reference memory is the same or the property members and their values are the same
Definition of Equality
Equality is defined as the data structure and property values are equivalent.
Same-value-zero
Numerical equivalence is based on Same-value-zero.
That is, all of the following comparisons are considered equivalent.
equal(NaN, NaN) // true
equal(0, 0) // true
equal(+0, 0) // true
equal(-0, 0) // true
equal(+0, -0) // true
Built-in objects
The following objects work correctly.
Array
Typed Array
(Int8Array
,Uint8Array
,Uint8ClampedArray
,Int16Array
,Uint16Array
,Int32Array
,Uint32Array
,Float32Array
,Float64Array
,BigInt64Array
,BigUint64Array
)ArrayBuffer
Object
Date
Error
(EvalError
,RangeError
,ReferenceError
,SyntaxError
,TypeError
,URIError
,AggregateError
)RegExp
Map
Set
URL
URLSearchParams
String
Number
Boolean
Do not guarantee the behavior of objects not on this list.
💚 Supports
ie is no longer supported to reduce bundle size.
The TypeScript version must be 4.1.0
or higher.
This project provides ES modules
and Commonjs
.
If you have an opinion about what to support, you can open an issue to discuss it.
The browserslist
has the following settings.
defaults
last 8 version
not IE <= 11
not ie_mob <= 11
node 6
Deno |
Node.js |
![]() Edge |
![]() Firefox |
![]() Chrome |
![]() Safari |
![]() iOS Safari |
![]() Samsung |
![]() Opera |
---|---|---|---|---|---|---|---|---|
^1.6.0 |
^6.17.0 |
^83 |
^78 |
^83 |
^11 |
^12.0 |
^7.2 |
^68 |
💫 Usage
equal
provides multi platform modules.
🦕 Deno
deno.land
import { equal } from "https://deno.land/x/equal/mod.ts";
equal([1, 2, 3], [1, 2, 3]); // true
nest.land
import { equal } from "https://x.nest.land/equal/mod.ts";
equal([1, ['hello', ['world']], [1, ['hello', ['world']]); // true
📦 Node.js
NPM package name is
lauqe
.
Install
npm i lauqe
or
yarn add lauqe
ES modules
import { equal } from "lauqe";
equal(new Date('2000/1/1'), new Date('2000/1/1')); // true
Commonjs
const { equal } = require("lauqe");
equal(/hello/g, /hello/g); // true
🌐 Browser
The module that bundles the dependencies is obtained from skypack.
<script type="module">
import { equal } from "https://cdn.skypack.dev/lauqe";
console.log(equal(() => {}, () => {}); // true
</script>
🤝 Contributing
Contributions, issues and feature requests are welcome!
Feel free to check issues.
🌱 Show your support
Give a ⭐️ if this project helped you!
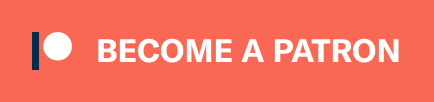
💡 License
Copyright © 2021-present TomokiMiyauci.
Released under the MIT license